Custom Request and Response objects in Express with TypeScript
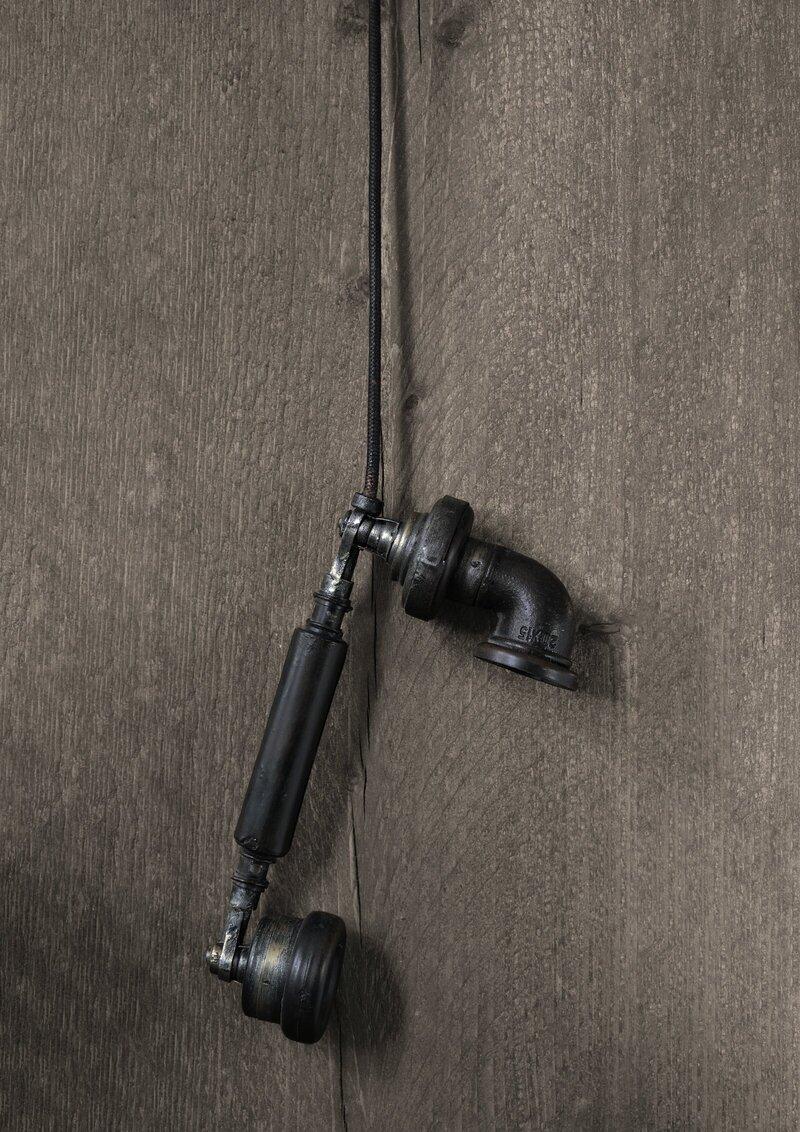
If you use TypeScript and Express you might want to put some custom properties on the Request
and Response
objects or have a custom User
object. This is how you do it.
Add type definitions
Add a folder called "type-definitions" to your project. In that folder create a file called "express.d.ts". This is where you will put your custom types.
import { CustomUser } from "../src/models/user.js";
declare global {
namespace Express {
// extend the built in User with your own custom properties
interface User extends CustomUser {}
// Extend the request and response objects with your own custom properties
export interface Request {
parsedForm?: SomeCustomType;
}
export interface Response {
locals: {
allowedRoles?: string[];
};
}
}
}
Tell typescript about your custom types
Now add the following to your tsconfig.json
file:
{
"compilerOptions": {
"types": ["./type-defs/express-request-merging.d.ts"]
}
}
Now you can use your custom types in your code
middleware(req:Express.Request, res:Express.Response) {
// req.parsedForm is now available
// res.locals.allowedRoles is now available
// req.user is now available with custom props
}